Template Simple Simulink¶
This template shows how to simulate a simple vehicle in Simulink using a s-function. The graphics are also plotted.
This model uses the s-function SimpleVehicleSFunction.m in Simulink. The package and this s-function must be in Matlab path.
The “SimpleVehicleSimulink.slx” available in the repository (“docs/examples/TemplateSimpleSimulink”) is illustrated below:
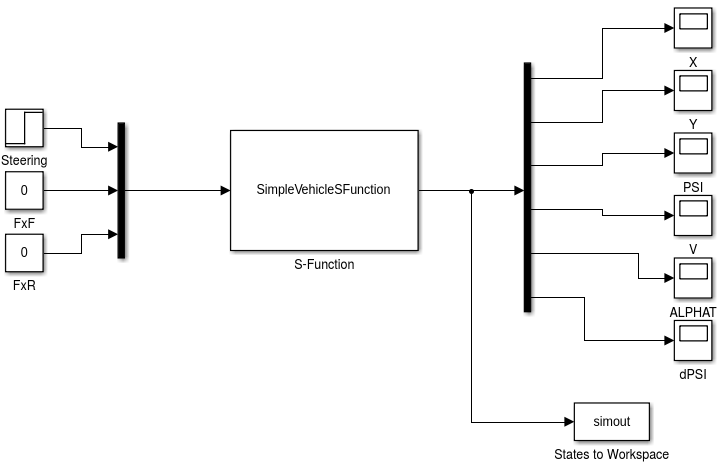
Fig. 59 Simple vehicle simulink S-Function
It can be seen that the longitudinal forces of the tire are zero for the entire simulation. The steering angle recieve a step input.
Template¶
To simulate the model run
sim('SimpleVehicleSimulink');
Each vehicle state variable goes to a scope. And the output of the model is saved in the workspace.
To generate the graphics, the same model used in Simple Vehicle S-Function must be defined.
Todo
Improve generation of graphics. Define parameters once -> Run Simulink -> Retrieve responses -> Graphics
First, define the tire model.
TireModel = TirePacejka(); % Choosing tire model
Defining the tire parameters to match the parameters used in the S-Function.
TireModel.a0 = 1;
TireModel.a1 = 0;
TireModel.a2 = 800;
TireModel.a3 = 3000;
TireModel.a4 = 50;
TireModel.a5 = 0;
TireModel.a6 = 0;
TireModel.a7 = -1;
TireModel.a8 = 0;
TireModel.a9 = 0;
TireModel.a10 = 0;
TireModel.a11 = 0;
TireModel.a12 = 0;
TireModel.a13 = 0;
The vehicle model is defined as
VehicleModel = VehicleSimpleNonlinear();
Defining the vehicle parameters to match the parameters used in the S-Function.
VehicleModel.mF0 = 700;
VehicleModel.mR0 = 600;
VehicleModel.IT = 10000;
VehicleModel.lT = 3.5;
VehicleModel.nF = 2;
VehicleModel.nR = 2;
VehicleModel.wT = 2;
VehicleModel.muy = .8;
VehicleModel.tire = TireModel;
Defining the simulation object.
simulator = Simulator(VehicleModel, tout);
Retrieving state responses from Simulink model
simulator.XT = simout.Data(:,1);
simulator.YT = simout.Data(:,2);
simulator.PSI = simout.Data(:,3);
simulator.VEL = simout.Data(:,4);
simulator.ALPHAT = simout.Data(:,5);
simulator.dPSI = simout.Data(:,6);
Frame and animation can be generated defining a graphic object (Graphics
). The only argument of the graphic object is the simulator object after the simulation.
g = Graphics(simulator);
To change the color of the vehicle run
g.TractorColor = 'r';
After that, just run
g.Frame();
g.Animation();
Both graphics feature can be seen below.
Todo
Include Template Articulated Simulink Animation
As expected the vehicle starts traveling in a straight line and starts a turn at (t = 1 , s) because of the step function.
Simple Vehicle S-Function¶
The simple vehicle S-Function is depicted below.
function [sys,x0,str,ts] = SimpleVehicleSFunction(t,x,u,flag)
% This file is a s-function template for simulating the simple vehicle model in Simulink.
% Choosing tire model
TireModel = TirePacejka();
% Defining tire parameters
TireModel.a0 = 1;
TireModel.a1 = 0;
TireModel.a2 = 800;
TireModel.a3 = 3000;
TireModel.a4 = 50;
TireModel.a5 = 0;
TireModel.a6 = 0;
TireModel.a7 = -1;
TireModel.a8 = 0;
TireModel.a9 = 0;
TireModel.a10 = 0;
TireModel.a11 = 0;
TireModel.a12 = 0;
TireModel.a13 = 0;
% Choosing vehicle model
VehicleModel = VehicleSimpleNonlinear();
% Defining vehicle parameters
VehicleModel.mF0 = 700;
VehicleModel.mR0 = 600;
VehicleModel.IT = 10000;
VehicleModel.lT = 3.5;
VehicleModel.nF = 2;
VehicleModel.nR = 2;
VehicleModel.wT = 2;
VehicleModel.muy = .8;
VehicleModel.tire = TireModel;
switch flag,
%%%%%%%%%%%%%%%%%%
% Initialization %
%%%%%%%%%%%%%%%%%%
case 0,
[sys,x0,str,ts]=mdlInitializeSizes();
%%%%%%%%%%%%%%%
% Derivatives %
%%%%%%%%%%%%%%%
case 1,
sys=mdlDerivatives(t,x,u,VehicleModel);
%%%%%%%%%%%
% Outputs %
%%%%%%%%%%%
case 3,
sys=mdlOutputs(t,x,u,VehicleModel);
%%%%%%%%%%%%%%%%%%%
% Unhandled flags %
%%%%%%%%%%%%%%%%%%%
case { 2, 4, 9 },
sys = [];
%%%%%%%%%%%%%%%%%%%%
% Unexpected flags %
%%%%%%%%%%%%%%%%%%%%
otherwise
DAStudio.error('Simulink:blocks:unhandledFlag', num2str(flag));
end
% end csfunc
%
%=============================================================================
% mdlInitializeSizes
% Return the sizes, initial conditions, and sample times for the S-function.
%=============================================================================
%
function [sys,x0,str,ts]=mdlInitializeSizes()
% Definitions
sizes = simsizes;
sizes.NumContStates = 6;
sizes.NumDiscStates = 0;
sizes.NumOutputs = 6;
sizes.NumInputs = 3;
sizes.DirFeedthrough = 1;
sizes.NumSampleTimes = 1;
sys = simsizes(sizes);
% Setting initial conditions
x0 = [0 0 0 20 0 0];
str = [];
ts = [0 0];
% end mdlInitializeSizes
%
%=============================================================================
% mdlDerivatives
% Return the derivatives for the continuous states.
%=============================================================================
%
function sys = mdlDerivatives(t,x,u,vehicle)
% Defining input
vehicle.deltaf = u(1);
vehicle.Fxf = u(2);
vehicle.Fxr = u(3);
% Getting the vehicle model function (state equations)
ModelFunction = @vehicle.Model;
sys = ModelFunction(t,x,0);
% end mdlDerivatives
%
%=============================================================================
% mdlOutputs
% Return the block outputs.
%=============================================================================
%
function sys=mdlOutputs(~,x,~,~)
% Output are all state variables
sys = x;
% end mdlOutputs